Run the following to create a workspace for your React components:
The generated workspace includes workspace-level configuration, configuration files for your IDE, and a customizable React Native env for your React Native component development.
To learn how to initialize a Bit workspace on an existing project, see Add Bit to an existing project.
Run the following to create a component using your env's react-native
template:
Component compilation
Run bit compile
to compile modified components, or watch mode (bit watch
) to auto-compile changes as they are made
Make your component as extensible as possible by passing all props native to the root element, using the ...rest
pattern. Enable custom styles using the className
prop.
Extensibility is key to maximizing component reuse.
/* @filename: welcome.tsx */ import { Text, StyleSheet, TextProps } from 'react-native'; export type WelcomeProps = { /** * a text to be rendered in the component. */ text: string } & TextProps; const styles = StyleSheet.create({ text: {}, }); export function Welcome({ text, ...rest }: WelcomeProps) { return ( <Text style={styles.text} {...rest} > {text} </Text> ); }
Expose your component's API and public types in the component's main file (index.ts
, by default):
/* @filename: index.ts */ export { Welcome } from './welcome.tsx'; export type { WelcomeProps } from './welcome.tsx';
Verify that your component behaves as expected by rendering it in various relevant contexts and variations.
For example, the following basic composition displays the pages/welcome
component when text = 'hello world!'
.
// @filename: welcome.compositions.tsx
import { Welcome } from './welcome';
export const BasicWelcome = () => {
return (
<Welcome text="Hello world!" />
);
}
You can add other compositions by exporting additional react functions with code examples using your component, from the same file or other *.composition.*
files.
Head to the component's 'Compositions' tab, to see your rendered compositions (run bit start
if you don't have the workspace UI running already).
Head over to your component's .spec.tsx
file to add automated testing.
We recommend using your compositions as the base for your testings. For example:
import { render } from '@testing-library/react-native'; import { BasicWelcome } from './welcome.composition'; it('renders with the correct text', () => { const { getByText } = render(<BasicWelcome />); const rendered = getByText('Hello world!'); expect(rendered).toBeTruthy(); });
See the Testing docs for more information.
Your bit components are just react-native code with bit's management layer on top. So you would install and use dependencies in exactly the same was as you would in any other react-native development environment.
To use a dependency, whether it's a component or a package, simply install it in your workspace, and import it into your component's files (components maintained in the same workspace do not need to be installed, but are nonetheless still consumed via their package name).
Bit will auto-detect this as a dependency for your component and add it to the component's package.json when you create a new snap or tag of your component.
To use this component as a dependency of another component, run
bit show pages/welcome
to see its package name. You'll notice that it's just@org/scope.name.spaces.component-name
. Use the package name to import it into other components.
Your component's documentation is automatically generated from documentation files you can add to the component, of the form *.docs.mdx
(this can be customised in your custom env's env.jsonc
file).
To add your own custom documentation, create a welcome.docs.mdx
documentation file inside the component directory.
MDX doc files use the MDX format.
For example:
---
description: 'A Welcome component.'
labels: ['label1', 'label2', 'label3']
---
import { Welcome } from './welcome';
## React Native Component for rendering text
A basic view that renders some text
### Component usage
```js
<Welcome text="hello from Welcome" />
```
### Using props to customize the text
Modify the text to see it change live:
```js live
<Welcome text="hello from Welcome" />
```
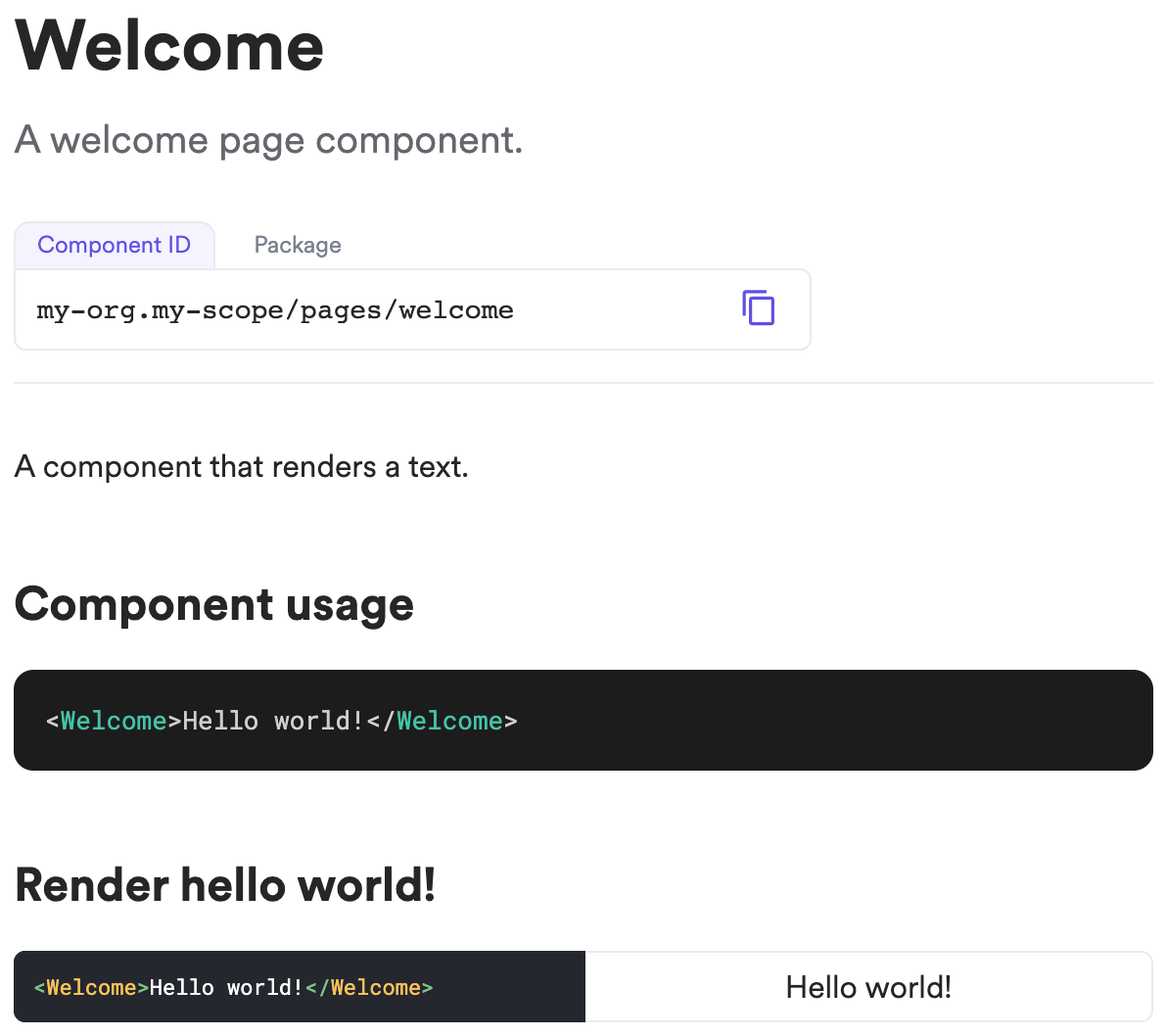
Snap your component and export it to its remote scope, to enable others to consume it and collaborate on it.